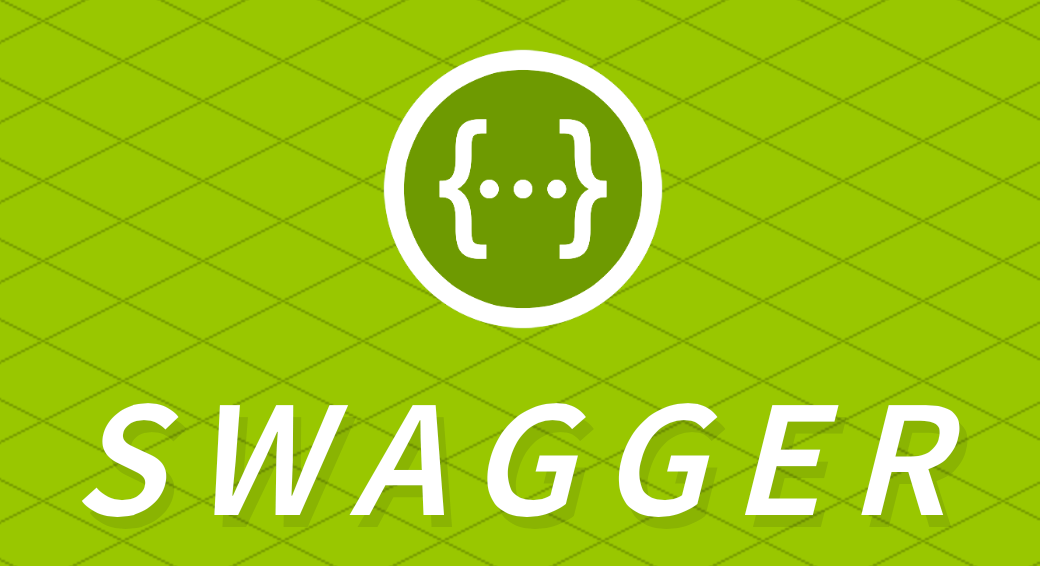
Documentation for REST services can quickly become outdated as code evolves. To address this challenge and keep service documentation up-to-date, an excellent tool called Swagger comes to the rescue. Swagger automatically generates and updates documentation, supporting formats like JSON or YAML.
With Swagger, you can easily create comprehensive and accurate documentation for your REST services. It captures essential information about your API, such as endpoints, request/response formats, headers, and parameters. Swagger also provides a user-friendly interface for exploring and testing your API directly from the documentation.
By leveraging Swagger, you can ensure that your API documentation stays in sync with your codebase, making it easier for developers and consumers to understand and interact with your REST services.
Used:
Swagger | Spring Boot | H2 In-memory Database | Spring Data JPA |
Need to perform:
- Implement a REST service on Spring Boot;
– Connect Swagger UI framework;
Using Spring Initializr is a convenient way to build a Spring project. Spring Initializr is a web-based service that provides a user-friendly interface for generating the initial structure of a Spring project with the necessary dependencies and configurations.
We define the project structure and implement a small REST service.
Next, you need to add a few dependencies to the pom.xml file.
io.springfox springfox-swagger2 2.9.2 io.springfox springfox-swagger-ui 2.9.2 |
Where artifactId springfox-swagger2 to connect JSON API documentation for Spring applications.
artifactId springfox-swagger-ui to be able to connect the UI interface.
Only one Bean with Swagger configuration needs to be added to the Spring Boot project:
@Configuration @EnableSwagger2 public class SwaggerConfig { @Bean public Docket api() { return new Docket(DocumentationType.SWAGGER_2) .select() .apis(RequestHandlerSelectors.any()) .paths(PathSelectors.any()) .build(); } } |
The Bean Docket
contains the configuration for Swagger 2, which can be both default and customizable.
Here’s a breakdown of the annotations and methods used:
@Configuration
: This Spring annotation informs the Spring container to treat the class as a configuration class, allowing for runtime configuration. It can be used with XML files as well.@EnableSwagger2
: This annotation enables Swagger 2 support in a Spring Boot application. It must be accompanied by the@Configuration
annotation.@Bean
: This standard Spring annotation creates and registers a component. In this case, it creates aDocket
bean for configuring the Swagger API documentation.Docket
: TheDocket
class is the basic API configuration mechanism for Swagger. It is initialized for the Swagger specification and provides default configuration options along with convenience methods for customization..select()
: This method returns an instance ofApiSelectorBuilder
, which allows you to manage the endpoints that Swagger will generate documentation for..apis()
: This method specifies the base package that will be scanned, and APIs will be created for all classes in it.
Here are a couple of examples:
.apis(RequestHandlerSelectors.any())
: This configures Swagger to generate documentation for all packages..apis(RequestHandlerSelectors.basePackage("ru.gotoqa.GameOfThronesService"))
: This restricts Swagger to theGameOfThronesService
package, generating documentation only for classes within that package.
The .paths()
method further specifies for which paths in our API we want to generate documentation.
Here are a couple of examples:
.paths(PathSelectors.any())
: This includes all endpoints in generating documentation..paths(regex("/version1.*"))
: This includes only the endpoints that match the pattern “/version1.*” in generating documentation..paths(Predicates.or(PathSelectors.regex("/version1.*"),PathSelectors.regex("/version2.*")))
: This restricts the documentation to multiple endpoints by using multiple path selectors.
These examples demonstrate how to customize the paths for which Swagger generates documentation. You can use different path selectors and patterns to include or exclude specific endpoints based on your requirements.
By default, Swagger provides a basic set of customization options to enhance your API documentation. Here are a few examples:
Through the .apiInfo(apiInfo()) method, you can add information about the owner, company etc.
private ApiInfo apiInfo() { return new ApiInfo( "REST API Game Of Thrones Service", "", "API 1.0", "http://gotoqa.ru/cv/", new Contact("Roman", "www.gotoqa.ru", "n@company.com"), "License of API", "http://gotoqa.ru", Collections.emptyList()); } |
String title, String description, String version, String termsOfServiceUrl, Contact contact, String license, String licenseUrl, Collection vendorExtensions |
Swagger Core. Doc’s
@Api | Помечает класс как Swagger resource. |
@ApiModel | Provides additional information about Swagger models. |
@ApiModelProperty | Adds and manipulates data of a model property. |
@ApiOperation | Описание HTTP метода |
@ApiParam | Adds additional meta-data for operation parameters. |
@ApiResponse | Describes a possible response of an operation. |
@ApiResponses | A wrapper to allow a list of multiple ApiResponse objects. |
@RestController //@RequestMapping("/gameofthrones") @Api(value="GameOfThronesService", description="Actor WEB Service") public class ActorController { @Autowired private ActorRepository actorRepository; @GetMapping("/version1/actors") @ApiOperation(value = "Returns list of all Actors") @ApiResponses(value = { @ApiResponse(code = 200, message = "Successfully retrieved list"), @ApiResponse(code = 401, message = "You are not authorized to view the resource"), @ApiResponse(code = 403, message = "Accessing the resource you were trying to reach is forbidden"), @ApiResponse(code = 404, message = "The resource you were trying to reach is not found") } ) List findAll(){ return actorRepository.findAll(); } @ResponseStatus(HttpStatus.CREATED) @PostMapping("/version1/actors") Actor newActor(@RequestBody Actor actor){ return actorRepository.save(actor); } @GetMapping("/version1/actors/{id}") @ApiOperation(value = "Returns Actor data") Actor findOne(@ApiParam("Id of the Actors to be obtained. Cannot be empty.") @PathVariable Long id) { return actorRepository.findById(id) .orElseThrow(() -> new ActorNotFoundException(id)); } |
The @ApiModelProperty annotation is used to describe the database model.
@Getter @Setter @Entity @NoArgsConstructor public class Actor { @Id @GeneratedValue @ApiModelProperty(notes = "The database generated actor's ID") private Long id; @ApiModelProperty(notes = "Actor first name", required = true) private String firstName; @ApiModelProperty(notes = "Actor second name", required = true) private String lastName; @ApiModelProperty(notes = "Actor birthDay", required = true) private String birthDay; public Actor(String firstName, String lastName, String birthDay) { this.firstName = firstName; this.lastName = lastName; this.birthDay = birthDay; } } |
Finally, an example of Swagger’s UI operation (/swagger-ui.html)
The examples provided in this article showcase the basic configuration and customization options of Swagger for documenting REST services. However, Swagger offers even more advanced functionalities, such as overriding standard HTTP method responses and implementing authorization. These features allow for greater control and customization of your API documentation. By exploring the full capabilities of Swagger, you can ensure that your API documentation remains up-to-date, comprehensive, and aligned with your evolving codebase.
Link to the full version of the project on GitHub:
Github GameOfThronesService